mssql 데이터베이스 연결
winForm을 이용해서 ms-sql과 데이터베이스를 연결하는 기본적인 내용
데이터베이스에 연결이후에는 여러가지 쿼리를 사용해서 데이터를 가져올수있다.
using System; using System.Collections.Generic; using System.Data.SqlClient; using System.Windows.Forms; namespace mssql_connect { public partial class Form1 : Form { private string _dbConnect; public Form1() { InitializeComponent(); } private void btn_connect_Click(object sender, EventArgs e) { string dbHost = txt_host.Text; string dbName = txt_name.Text; string dbId = txt_id.Text; string dbPass = txt_pass.Text; if (string.IsNullOrWhiteSpace(dbHost)) { MessageBox.Show("서버 아이피를 입력해주세요"); txt_host.Focus(); } else if (string.IsNullOrWhiteSpace(dbName)) { MessageBox.Show("데이터베이스 이름을 입력해주세요"); txt_name.Focus(); } else if (string.IsNullOrWhiteSpace(dbId)) { MessageBox.Show("계정 아이디를 입력해주세요"); txt_id.Focus(); } else if (string.IsNullOrWhiteSpace(dbPass)) { MessageBox.Show("계정 패스워드를 입력해주세요"); txt_pass.Focus(); } else { var dbConnectText = new List { string.Concat("Data Source=", dbHost), string.Concat("Initial Catalog=", dbName), string.Concat("User ID=", dbId), string.Concat("Password=", dbPass) }; _dbConnect = string.Join(";", dbConnectText); using (var conn = new SqlConnection(_dbConnect)) { try { SqlCommand cmd = new SqlCommand(); cmd.Connection = conn; cmd.CommandText = "SELECT GETDATE()"; conn.Open(); SqlDataReader rdr = cmd.ExecuteReader(); if (rdr.Read()) { this.result.Text = "연결 성공"; } else { this.result.Text = "연결 실패"; } } catch (Exception) { return; } finally { if (conn != null) { conn.Close(); } } } } } } }
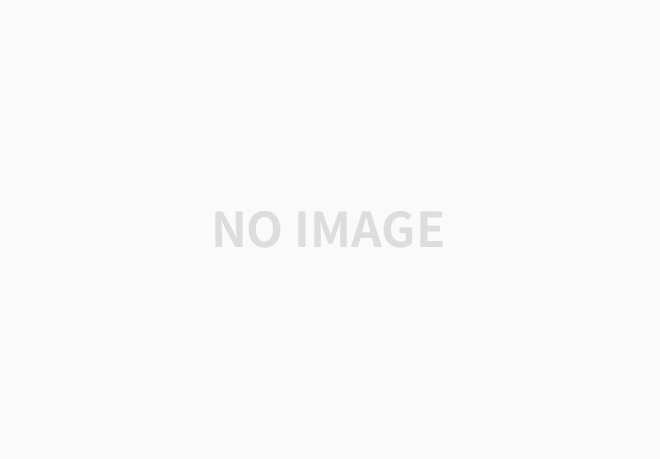
'C# > winForm' 카테고리의 다른 글
바인딩소스 (BindingSource) (0) | 2021.02.19 |
---|
댓글을 사용할 수 없습니다.